Curious about D language programming? D is a powerful and flexible language designed to make both simple coding and complex system programming more efficient. This guide covers D’s unique features, versatility across different programming paradigms, robust memory management, and seamless integration with other languages. Explore how D can benefit your projects.
Table of Contents
Key Takeaways
D programming language boasts key features such as closures, anonymous functions, compile-time function execution, and built-in container iteration, enhancing its flexibility and performance.
D supports multiple programming paradigms including concurrent, object-oriented, imperative, and functional programming, making it a versatile tool for diverse projects.
D Language Foundation plays a pivotal role in supporting and advancing the D programming language through community involvement, funding, and scholarships, ensuring its ongoing development and improvement.
Key Features of D Language
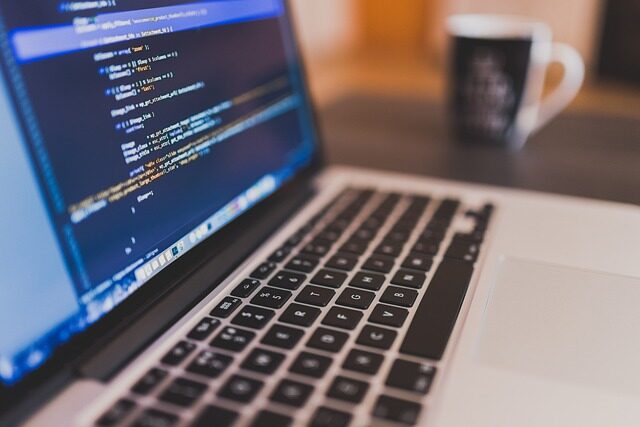
D is a potent and flexible programming language, thanks to its rich set of features. One of the standout features is its support for closures, which allow functions to capture and retain access to variables from their surrounding scope. This capability is particularly useful for creating more flexible and powerful function definitions, as it enables functions to remember the context in which they were created.
D’s support for anonymous functions is another feature worth noting. These functions can be defined without a name, using both longhand and shorthand syntax, which makes the code more concise and improves readability. This feature is especially beneficial in scenarios where a function is used only once or is passed as an argument to higher-order functions.
The ability to execute functions at compile time (CTFE) stands as one of D’s key strengths. This capability enhances the language’s flexibility and performance. CTFE allows certain calculations to be performed during the compilation process, optimizing runtime performance and enabling advanced metaprogramming capabilities. This feature can significantly reduce the runtime overhead and make the D source code more efficient.
Additionally, D shines in supporting ranges and built-in container iteration.
Ranges provide a unified interface for iterating over sequences, simplifying the implementation of generic algorithms.
D includes built-in container iteration concepts like the foreach loop, which simplifies the process of traversing various data structures.
Combined with type inference, which allows the compiler to automatically deduce the types of variables and expressions, D reduces boilerplate code and enhances readability.
Programming Paradigms Supported by D
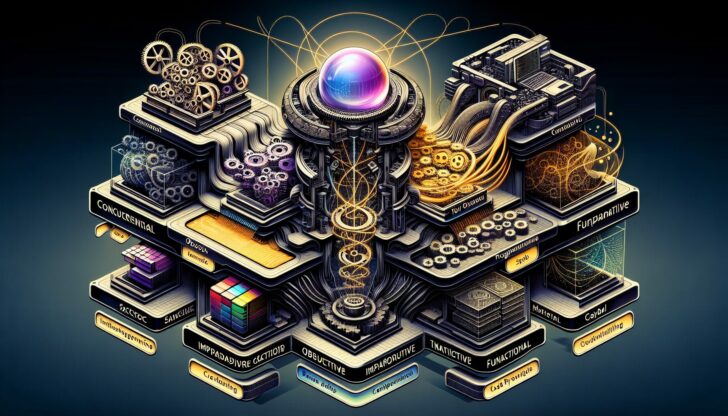
With its wide array of supported programming paradigms, D proves to be a versatile tool for diverse projects. One of the primary paradigms supported by D is concurrent programming, which utilizes the actor model to simplify the development of concurrent applications. This model allows developers to manage concurrent processes more efficiently, making D an excellent choice for applications requiring high levels of concurrency.
In addition to concurrent programming, D supports object-oriented programming, which uses a single inheritance hierarchy with Java-style interfaces and mixins. This approach allows for the creation of modular and reusable code, which is a hallmark of modern software development practices. D’s object-oriented features are designed to be intuitive and powerful, enabling developers to build complex systems with ease.
D, a general purpose programming language, also provides substantial support for imperative and structured programming, as well as system programming language features. Features such as the foreach loop and nested functions, which are distinct from those in other programming languages like C, make imperative programming in D more efficient and expressive. These features facilitate the development of clear and maintainable code, which is crucial for long-term project success.
D’s prowess is further exhibited in the paradigm of functional programming, including language features such as function literals, closures, and higher-order functions. These capabilities allow developers to write more concise and expressive code, leveraging the power of functional programming to create robust and maintainable applications. The combination of these paradigms within a single language makes D a highly adaptable and powerful tool for developers.
Memory Management in D
D provides a robust approach to the critical aspect of programming – memory management, by supporting both garbage collection and manual methods. D’s garbage collection system periodically returns unused memory to the pool of available memory, which can lead to faster program execution by eliminating the overhead of explicit memory allocation and deallocation. This feature is particularly beneficial for long-running applications where memory leaks can cause significant issues over time.
Fine-tuning of D’s garbage collector is possible through command line options, environment variables, or options embedded in the executable. This flexibility allows developers to optimize memory management based on the specific needs of their applications. Additionally, D includes precise heap scanning, which uses type information to identify actual pointers within heap-allocated data, enhancing the accuracy of garbage collection.
D’s garbage collector also boasts of an advanced feature – parallel marking. By utilizing all available CPU cores, the garbage collector can perform marking operations more efficiently, which can be configured by setting the ‘parallel’ GC option. This capability is particularly useful for applications running on multi-core systems, where efficient use of resources is crucial.
D provides mechanisms for those developers who prefer manual memory management and wish to write code without the garbage collector’s involvement. Functions like core.stdc.stdlib.malloc() and core.stdc.stdlib.free() allow explicit memory allocation and deallocation. Additionally, D includes features like the return and scope function parameter attributes, which help track and manage low-level pointers passed to functions, ensuring memory safety.
Overall, D’s memory management capabilities provide a balance between automated and manual control, making it suitable for a wide range of applications from high-performance system programming to large-scale applications where memory stability is paramount.
SafeD: Ensuring Memory Safety
SafeD, D’s approach to ensuring memory safety, serves as a cornerstone of reliable software. SafeD enforces compile-time checks on functions marked as @safe or @trusted, ensuring that operations within these functions are memory-safe. This proactive approach helps prevent common memory-related errors, such as buffer overflows and pointer arithmetic issues, which can lead to unpredictable behavior and security vulnerabilities.
In terms of memory safety, D classifies functions into three categories: @safe, @trusted, and @system. @safe functions have strict restrictions to prevent operations that may cause memory corruption. For example, they cannot break the type system through casts or modify pointer values in unsafe ways. @trusted functions can be called from @safe functions and have capabilities similar to @system functions, but they are expected to be used sparingly and with caution.
To enforce memory safety, array bounds checks are activated by default for @safe code, even in release mode. This feature ensures that array accesses are within bounds, preventing common programming errors that can lead to crashes or data corruption. Additionally, memory safety protections are available even in the BetterC subset of D, highlighting the language’s commitment to safe code execution in all environments.
Interoperability with Other Languages
D’s significant strength lies in its interoperability with other languages, facilitating seamless integration into existing projects. One of the most notable features is D’s ability to call C functions directly without requiring wrapper functions, allowing it to work with native code efficiently. By declaring C functions with a calling convention like extern (C), D can easily interface with C APIs, facilitating the integration of existing C libraries into D projects.
The ImportC compiler extension in D extends this interoperability, enabling direct import or compilation of .c files. This feature simplifies the process of incorporating C code into D projects, making it easier for developers to leverage existing C codebases. Furthermore, bindings for popular C libraries are available in D’s standard library and package repository, providing ready-to-use interfaces for a wide range of functionalities.
D also aligns and packs structs and unions similar to C, employing explicit alignment attributes. This compatibility extends to accessing C globals using the extern __gshared storage class, ensuring seamless interaction between D and C at a low level. Additionally, C code can call D functions if they are declared with a compatible attribute such as extern (C). These features collectively make D a versatile choice for projects that require tight integration with C or other languages.
Better C Subset
BetterC, a subset of the D programming language, simplifies integration with C projects without compromising most of D’s powerful features. Enabling BetterC is as simple as setting the -betterC command line flag, which activates the predefined version D_BetterC for conditional compilation. This feature allows developers to write D code that can be easily embedded within larger C projects without the overhead of initializing the D runtime.
Supplying a C main() function allows for an entire program to be written in BetterC, thereby further streamlining the integration process at the build-system level. BetterC retains nearly the full language, including compile-time features, metaprogramming, and nested functions, making it a powerful tool for developers who need the advanced capabilities of D without the runtime dependencies.
Nonetheless, BetterC lacks some features, including garbage collection, TypeInfo, and dynamic arrays. These omissions are intentional to reduce the complexity and increase the predictability of the resulting code when integrating with C. Despite these limitations, BetterC provides a streamlined and efficient way to leverage D’s capabilities in C-centric projects.
History and Evolution of D
D programming language, developed by Walter Bright in 1999, boasts a rich history. D 1.0 was officially released in 2007, marking a significant milestone in the language’s evolution. This initial release introduced many of the features that set D apart from other languages, including its unique combination of low-level control and high-level abstractions.
On June 17, 2007, D 2.0 was unveiled, further refining and expanding the capabilities of the language. The publication of ‘The D Programming Language’ by Andrei Alexandrescu in 2009 provided a comprehensive guide to the language, helping to establish its theoretical foundations and practical applications. Another significant publication, ‘Programming in D: Tutorial and Reference’ by Ali Çehreli, was released in 2015, offering additional insights and guidance for developers.
The establishment of the D Language Foundation has played a crucial role in backing the language’s development and promoting its adoption within the programming community. The Foundation’s efforts have included rewriting the D frontend in D to improve compilation times and overall performance. These milestones highlight the ongoing evolution of D, driven by a combination of community involvement and dedicated development efforts.
Implementations and Platforms
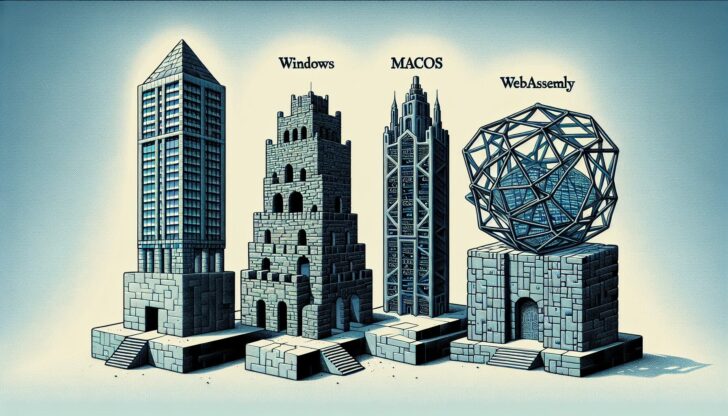
D showcases its versatility with its capability to be directly compiled into machine code for a variety of architectures and platforms. Integrated into GCC, the GDC compiler enables D code compilation alongside other supported languages such as C and C++. This integration provides a seamless workflow for developers who work with multiple languages within the same project. Additionally, the digital mars d compiler offers another option for those looking to work with D language.
LDC, another notable D compiler, utilizes the LLVM backend and supports an extensive range of architectures and operating systems. This compiler is known for its performance and flexibility, making it a popular choice for developers targeting different platforms. D supports platforms such as:
Windows
Linux
macOS
FreeBSD
Android
This ensures that developers can deploy their applications across various environments.
Besides traditional platforms, D can target WebAssembly using the LDC compiler, facilitating execution in web browsers and other Wasm environments. This capability opens up new possibilities for web development and other applications where WebAssembly’s performance and portability are advantageous.
D’s support for multiple architectures, including IA-32, AMD64, AArch64, PowerPC, MIPS64, DEC Alpha, Motorola m68k, SPARC, and s390, further underscores its versatility and broad applicability.
Development Tools for D Programmers
A rich ecosystem of development tools is accessible to developers working with D, designed to boost productivity and streamline the process of writing code. One notably popular tool is Code-d for Visual Studio Code, offering support for DUB integration, code completion, code formatting, static linting, and debugging with gdb or mago-mi. This extension transforms VS Code into a powerful IDE for D, making it easier for developers to write and debug their D code.
Visual-D is another valuable tool, offering a Visual Studio plugin that provides comprehensive support for D language development. This plugin includes features such as code completion, building, and debugging support, making Visual Studio a robust environment for D programmers. The IntelliJ IDEA Plugin for D adds similar functionality to IntelliJ IDEA, including DUB support, code completion, code formatting, linting, and debugging with gdb or mago-mi.
For those seeking a dedicated IDE, Dexed is an excellent choice. Dexed supports compilers like DMD, GDC, and LDC, and integrates tools such as DUB, DCD, D-Scanner, and Dfmt. This IDE is tailored specifically for D programming, offering features that cater to the unique needs of D developers. Additionally, D-Velop Extension for Nova integrates D via the Serve-D Language Server and uses a Tree-sitter D grammar, providing another robust option for D development.
D programmers, working with one of the many programming languages, also have access to powerful debugging tools. For instance, Dlang IDE, written in D itself, supports DUB, syntax highlighting, code completion with DCD, and debugging with GDB, mago-mi, or lldbmi2. Zeus, a language-neutral programmer’s editor/IDE for Windows, supports accurate D syntax highlighting, further expanding the toolkit available to D developers.
Practical Examples
Practical examples are invaluable for understanding how a programming language performs in real-world scenarios. D’s design eliminates undefined and implementation-defined behaviors, providing a stable environment for practical programming. Two such examples that showcase D’s capabilities are handling command line arguments and detecting anagrams.
Command line arguments are a common requirement in many applications, and D makes it straightforward to handle them using the main function and foreach loops. This example will demonstrate how D’s syntax and features simplify this task, making it efficient and user-friendly.
Anagram detection, a classic text processing problem, can be effectively tackled using D’s built-in associative arrays and data manipulation capabilities. This example will highlight how D’s powerful features enable quick and efficient implementation of complex algorithms, making it ideal for tasks such as text processing and machine learning.
Command Line Arguments
Handling command line arguments in D is both simple and elegant. In D, command line arguments can be accessed using the main function with the signature void main(string[] args). This function receives command line arguments as an array of strings, allowing easy access to the parameters passed to the program.
To process these arguments, D provides the foreach loop, which allows straightforward iteration over the command line arguments. This feature simplifies the task of handling input, making it easy to parse and use command line parameters within the program’s logic.
Anagram Detection
Anagram detection is an excellent example of D’s capabilities in text processing. By using D’s built-in associative arrays, developers can efficiently implement anagram detection algorithms. Associative arrays allow for quick and easy grouping of words with the same sorted character sequences, which is the key to identifying anagrams.
This technique, which involves counting character frequencies and comparing them, highlights D’s powerful data manipulation capabilities and its suitability for tasks that require efficient handling of large datasets.
Real-World Applications of D
Notable companies employ the D programming language in diverse projects, showcasing its versatility and reliability. Facebook, eBay, and Netflix are among the prominent organizations that have adopted D for their development needs. These companies leverage D’s performance and flexibility to build robust, scalable applications.
In the development of AAA games, where performance and efficiency are paramount, D is also put to good use. The language’s powerful features and high-level abstractions make it an ideal choice for game development, allowing developers to focus on creating immersive experiences without sacrificing performance.
D also finds use in text processing, web and application servers, and machine learning. These applications benefit from D’s ability to handle complex data manipulations and high-throughput requirements, showcasing the language’s broad applicability across different domains.
Critique and Community Feedback
The D programming language has encountered critiques, particularly concerning its error correction process. In 2024, a fork named OpenD was created to address these concerns, aiming to cater to the community’s demands for a more efficient error correction process. This move sparked discussions about governance models and the importance of balancing stakeholder requirements.
The introduction of OpenD was driven by longstanding frustrations within the community regarding the slow adoption of language improvements. Some community members believe that poor governance and leadership contributed to the need for this fork. The decision to create OpenD reflects a desire for more agile and responsive development practices.
The community has exhibited mixed reactions to OpenD. While some members support the move for better error handling and see it as a necessary evolution, others are skeptical about its future. There are concerns that the fork might further fragment the D programming community and dilute its mindshare.
Despite the challenges, the discussions around OpenD have underscored the community’s fervor and commitment towards advancing the D language. Whether OpenD will succeed in revitalizing the language remains to be seen, but it has undeniably sparked important conversations about governance and development practices within the community.
Support and Development
The D Language Foundation assumes a crucial role in backing and coordinating the development of the D programming language. Established to promote and advance D, the Foundation oversees various initiatives aimed at improving the language and fostering community involvement. In 2016, the Foundation became a tax-exempt non-profit organization, dedicated to advancing open-source technology related to D.
Through events, forums, and collaborative projects, the Foundation fosters community involvement. Volunteers can contribute to the development, documentation, testing, and community outreach efforts, helping to drive the language forward. Contributors to D’s source code are recognized by the Foundation, highlighting the collaborative nature of D’s development.
Monetary donations significantly contribute to supporting D’s development. Donations help fund various aspects of the language’s growth, including tooling, documentation, and community events. The Foundation maintains a list of sponsors who contribute financially, ensuring transparency and fostering a sense of collective ownership within the community.
Further promoting the study and development of the D language as a primary language, the Foundation offers Languages and Systems Research Scholarships to Electrical Engineering and Computer Science students at the University ‘Politehnica’ Bucharest (UPB). These scholarships support the next generation of developers and researchers, ensuring the continued evolution of D.
Learn more, 5 reasons the D programming language is a great choice for development.
Summary
The D programming language stands out for its powerful features, versatile programming paradigms, and robust memory management. From closures and anonymous functions to compile-time function execution and ranges, D offers a rich set of tools that enhance developer productivity and code quality. Its support for multiple programming paradigms, including concurrent, object-oriented, imperative, and functional programming, makes it a highly adaptable language suitable for various applications.
The strong community support, facilitated by the D Language Foundation, ensures ongoing development and improvement of the language. Despite some critiques and challenges, the passion and commitment within the D community continue to drive the language forward. Whether you are working on high-performance systems, web servers, or machine learning projects, D provides the tools and flexibility to achieve your goals efficiently and effectively.
Frequently Asked Questions
What are the key features of the D programming language?
The key features of the D programming language include closures, anonymous functions, compile-time function execution (CTFE), ranges, built-in container iteration, and type inference. These features make D a powerful language.
How does D ensure memory safety?
D ensures memory safety through its SafeD approach, which enforces compile-time checks on functions marked as @safe or @trusted, preventing memory corruption and ensuring safe code execution.
What programming paradigms does D support?
D supports multiple programming paradigms, such as concurrent, object-oriented, imperative, functional, and metaprogramming, offering versatility for various practical applications.
How does D handle memory management?
D offers both garbage collection and manual memory management options, allowing developers to choose the best approach for their specific needs. Garbage collection periodically returns unused memory, while manual memory management can be achieved using functions like core.stdc.stdlib.malloc() and core.stdc.stdlib.free().
What is BetterC in the D programming language?
BetterC in the D programming language is a subset that simplifies integration with C projects by retaining most features of D while excluding garbage collection, TypeInfo, and dynamic arrays. It can be enabled using the -betterC command line flag.